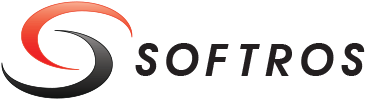
Softros LAN Messenger SDK (Software Development Kit) provides headers, libraries, and examples for embedding message sending functionality to your software. The SDK requires:
Softros LAN Messenger provides access to its APIs via MessengerAPIx86.dll and MessengerAPIx64.dll dynamic link libraries for x86 and x64 architectures. The ARM architecture is not supported. Libraries are included in Softros LAN Messenger installation and located in \SDK\DLLs\ subfolder. Usage examples in C#, C++, and Delphi programming languages can be found in \SDK\DLLs\Examples\ subfolder.
Currently, SDK provides two functions for that:
uint SendMessage( recipientTypeEnum recipientType, string recipientValue, string MsgText );
DWORD SendMessage( [in] recipientTypeEnum recipientType, [in] const wchar_t* recipientValue, [in] const wchar_t* MsgText );
function
SendMessage(recipientType: DWORD; recipientValue: PWideChar; MsgText: PWideChar): DWORD;
stdcall;
uint SendFiles( recipientTypeEnum recipientType, string recipientValue, string MsgText, string FilesList );
DWORD SendFiles( [in] recipientTypeEnum recipientType, [in] const wchar_t* recipientValue, [in] const wchar_t* MsgText, [in] const wchar_t* FilesList );
function
SendFiles(recipientType: DWORD; recipientValue: PWideChar; MsgText: PWideChar; FilesList: PWideChar): DWORD;
stdcall;
recipientType
[in] recipientType
recipientType:
public enum recipientTypeEnum { UID = 0, IP = 1, PCName = 2, UserName = 3, Group = 4, All = 5 }
enum class recipientTypeEnum { UID = 0, IP = 1, PCName = 2, UserName = 3, Group = 4, All = 5 };
//cast to DWORD
type
TrecipientTypeEnum = (UID, IP, PCName, UserName, Group, All);
The type of recipient. See Recipient Types for details.
string recipientValue
[in] const wchar_t* recipientValue
recipientValue: PWideChar
The recipient. The format depends on the value of the recipientType. See
Recipient Types for details.
Can be nullNULLnil
only if the value of recipientType is All.
string MsgText
[in] const wchar_t* MsgText
MsgText: PWideChar
The message text. Can be NULL only when used with SendFiles function and
if no message needs to be attached to the file transfer.
string FilesList
[in] const wchar_t* FilesList
FilesList: PWideChar
The list of paths to files and/or folders separated by line breaks, i.e.,
"\r\n"L"\r\n"#13#10
If the function succeeds, the return value is zero. If the function fails, the return value is one of the following error codes:
Code | Description | Possible remedy |
---|---|---|
1 | Error: The recipient has not been found. | Wrong name of the recipient. Please double-check its UID, name or address along with case sensitivity where applicable. |
2 | Error: The SDK API is not enabled in the Admin.ini. | Enable it by setting the AllowSDKAPI parameter to 1. |
3 | Error: Softros LAN Messenger is not running. | Check if Softros LAN Messenger is installed and running |
Successful execution of the function does not guarantee the message has been received by the recipient. For example, in case the recipient is offline, the message is stored for later delivery.